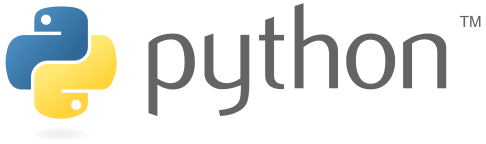
Python socket library is powerful and can be for socket based data transfer for both TCP and UDP protocol. This tutorial with TCP. I have created two modules. One tcpServer.py and other is tcpClient.py.
1. tcpServer.py
This module simply bind the socket object to the server ipaddress to a particular port.
Then it's listen for incoming connection from the client. It does nothing until it receives any
request. Once it receive request it accepts and enter into data exchange (chat). The server does
nothing but receives data send by the client and send back the same to client converting it to
uppercase. If the client terminates the server again go back to the state where it again listen for
some new connection request. Server can be stopped by pressing Ctrl+C. The program is included
inside Main() function to make it executable only. Cannot be used as library in any other module.
tcpServer.py code
import socket # Socket library
def Main(): # main function
host = "127.0.0.1" # host loop back address to test in adapter-less machine.
port = 5000 # port for server
s = socket.socket() # socket object.
s.bind((host,port)) # binding to server ip and port mentioned.
while True: # infinite loop ends on Ctrl+C by user.
print "Server is waiting for connection...." # waiting message displayed
s.listen(1) # listening for incoming connection request.
c, addr = s.accept() # Connection request accepted and connection object and client ip address
# returned
print "Connection accepted from: " + str(addr) # message printed for connection success
while True: # infinite loop to exchange message with client
data = c.recv(1024) # receiving if any data send by client
if not data: # checking if data exists, this condition is only false when
break # client program terminates.
print "Data recieved from user: " + str(data)
print "Sending data to client"
data = str(data).upper() # received data converted to uppercase.
c.send(data) # received data send to client
c.close() # connection closed.
if __name__ == '__main__': # Main is not called automatically but with this statement.
Main()
2. tcpClient.py.
TCP client takes host ip and host port to connect (server binds and client connects). It asks input
from user (message) and it is send to the server until user enters quit in input.
import socket
def Main():
host = "127.0.0.1"
port = 5000
s = socket.socket()
s.connect((host,port)) # Connects to server host and port.
message = raw_input("==>") # Input from user.
while message!= "quit": # Loop until user enters quit
s.send(message) # Entered message send to server.
data = s.recv(1024) # Received data from server as response.
print "Message from server: " + str(data) # print the received message
message = raw_input("==>") # Waits for the next user input
s.close() # socket closed.
if __name__ == '__main__':
Main()
------------------------------------------------------------------------------------------------------------------------------------
#python #pythoprogramming #pythoscript #socketprogramming
No comments:
Post a Comment